Last Updated on July 10, 2024
Groovy Language
Groovy is designed to seamlessly integrate with the Java platform. Optional static typing i.e. Dynamic typing in Groovy offers a unique blend of familiarity and flexibility, empowering developers to write cleaner, more concise, and ultimately more maintainable code.
One of Groovy’s defining characteristics is its optional typing system. Unlike Java’s strict static typing, Groovy allows developers to choose between explicitly defining variable types (static typing) or letting the language infer them at runtime (dynamic typing). This flexibility caters to different development needs.
For tasks requiring strong type safety and performance optimization, static typing remains an option. However, for rapid prototyping or scripting tasks, dynamic typing allows for a more agile approach.
Static Typing vs. Dynamic Typing
Static typing lies in the concept of early error detection.
By requiring developers to define variable types at compile time, the compiler performs a rigorous check for type compatibility between variables and expressions. This acts as a safety net, catching potential errors like trying to add a string to an integer before the program even runs.
By explicitly declaring variable types, statically typed languages enhance the clarity and purpose of the code.
This can sometimes make the code more verbose compared to dynamically typed languages and it can be a minor inconvenience for simple scripts, but in larger projects, the extra verbosity might become noticeable and potentially affect developer productivity.
As opposed to static typing, dynamic typing is an approach to programming languages where the data type of a variable is determined at runtime.
Since there’s no need to explicitly declare variable types, dynamic typing can sometimes lead to more concise code compared to static typing. This can be beneficial for simple scripts or when readability isn’t a major concern.
The lack of upfront type checking in dynamic languages can lead to errors that only manifest during runtime. This can make debugging more challenging compared to static typing where errors are caught earlier in the development process.
Dynamic typing offers advantages in terms of flexibility, conciseness, and potentially faster development cycles. However, it’s important to consider the trade-offs in terms of readability and potential runtime errors when choosing a language for your project.
Groovy Optional Static Typing
To Demonstrate features we can create a maven groovy project in IntelliJ IDEA
If we create two different classes Circle and Square with same method name getArea()
public class Circle {
int radius =1;
double pi = 3.14;
double getArea(){
return radius*radius*pi;
}
}
public class Square {
int side =1;
double getArea(){
return side*side;
}
}
In the main class we define a list containing the two objects:
static void main(String[] args) {
Circle circle = new Circle();
Square square = new Square();
def list = [circle, square]
list.each { obj ->
println obj.getArea();
};
}
If we run the program we see that it calcuates areas without error:
3.14
1.0
Process finished with exit code 0
Demonstrating how Groovy does not require compile time type checking by default.
Lets look at another example where we have a class named MathUtils:
class MathUtils {
static int add(int a, String b) {
return a + b
}
}
For the code below, Groovy does not give any error in compile time
static void main(String[] args) {
def result = MathUtils.add(5, 5)
}
However when we run the program we get following runtime error.

To eliminate this type of inconveniences we can force compile time type checking in a class or method in Groovy.
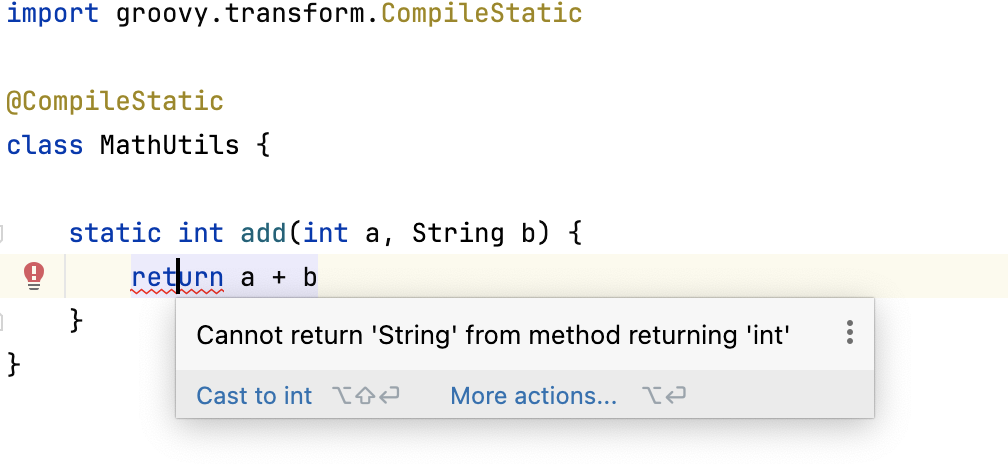
Now we are not allowed to compile the code and the error is caught in development phase.
@CompileStatic
offers granular control over static type checking in Groovy. You can choose to enforce it for entire classes or just specific methods, depending on your needs.