Last Updated on June 27, 2024
What is Blockchain?
Oxford Dictionary defines blockchain as:
“A system in which a record of transactions, especially those made in a cryptocurrency, is maintained across computers that are linked in a peer-to-peer network”.
Blockchain is a decentralized ledger of information. It consists of blocks of data connected through the use of cryptography. It belongs to a network of nodes connected over the public network.
The term ledger denotes a database. We can say that blockchain is a database technology that stores data in distributed decentralized manner.
In a distributed network, all participants have access to the distributed ledger(database) and its immutable record of transactions.
All nodes in the network work autonomously and manipulation of data records are prevented by proof of work algorithm.
No participant can change or tamper with a transaction after it’s been recorded to the shared ledger, this property is know as immutability of records, .
As each transaction occurs, it is recorded as a “block” of data. Those transactions show the movement of an asset that can be tangible (a product) or intangible (intellectual).
These blocks form a chain of data as an asset moves from place to place or ownership changes hands, removing the possibility of tampering by a malicious actor, and builds a ledger of transactions you and other network members can trust.
To speed transactions, a set of rules that are called a smart contract is stored on the blockchain and run automatically. Smart contracts are digital contracts stored on a blockchain that are automatically executed when predetermined terms and conditions are met.
A smart contract defines conditions for corporate bond transfers, include terms for travel insurance to be paid and much more.
Blockchain is a group of inter-related technologies of cryptography, peer-to-peer protocols, distributed databases, anonymity etc. In addition to this blockchain is foundation of many other technologies built on top of it, most prominently cryptocurrencies.
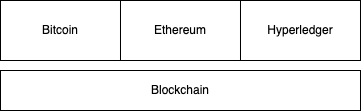
Blockchain development tools
Let’s go through some of the popular tools to work within this space:
Solidity is a statically-typed curly-braces programming language designed for developing smart contracts that run on the Ethereum Virtual Machine. Smart contracts are programs that are executed inside a peer-to-peer network where nobody has special authority over the execution, and thus they allow anyone to implement tokens of value, ownership, voting, and other kinds of logic.
Remix IDE is used for the entire journey of smart contract development by users at every knowledge level. It requires no setup, fosters a fast development cycle, and has a rich set of plugins with intuitive GUIs.
Part of Truffle suite, an Ethereum developer tool that allows you to simulate a blockchain environment locally and test deployed smart contracts. Ganache helps developers test and debug their applications before deploying them on a live blockchain network.
Go Ethereum is a command-line interface that gives developers the ability to process full Ethereum nodes, carry out smart contracts and mine the cryptocurrency. It is the official Ethereum client for building decentralized applications using the Go programming language. Geth is one of the preferred alternatives for running, setting up nodes, and interacting with Ethereum blockchains due to its ease of use.
Truffle is a popular development framework specifically designed to streamline the process of building decentralized applications (dApps) on blockchains that utilize the Ethereum Virtual Machine (EVM). By offering features like automated testing, network management, and an interactive console, Truffle simplifies the development workflow, saving developers time and effort.
Parity helps in setting up the development environment for smart contracts on Ethereum. It provides a fast and secure way to interact with the blockchain.
Java Blockchain Libraries
Web3j
Web3j is a lightweight, highly modular, reactive, type safe Java and Android library for working with Smart Contracts and integrating with clients (nodes) on the Ethereum network.
It allows developers to connect to Ethereum nodes, which are computers that store and validate data on the blockchain. Through this connection, developers can then perform actions on the blockchain, such as sending transactions or deploying smart contracts
BitcoinJ
Unlike Web3j, which focuses on Ethereum, BitconiJ is a library designed specifically for working with the Bitcoin blockchain. It’s likely another tool for developers, similar to Web3j for Java, but aimed at interacting with Bitcoin. This library is very useful for anyone who wants to understand how the Bitcoin protocol works.
HyperLedger Fabric
The Fabric Gateway is a core component of a Hyperledger Fabric blockchain network, and coordinates the actions required to submit transactions and query ledger state on behalf of client applications.
Blockchain in Java
To demonstrate simple blockchain application in Java we can create a maven project in IntelliJ IDEA. For this application we don’t need to add any library for block chain. We only add dependencies for sl4j logging, lombok and Junit testing.
Basic fundamental unit in blockchain is Blok. Blok contains one or more transactions and it has a hash value to make it tamperproof.
Calculating a hash value is computationally intensive and its called “mining”.
A block is added to the network by consensus of all nodes.
Lets begin:
package org.example;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.UnsupportedEncodingException;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.logging.Level;
import static java.nio.charset.StandardCharsets.UTF_8;
@Getter
@Setter
@NoArgsConstructor
public class Block {
Logger logger = LoggerFactory.getLogger(Block.class);
private String hash;
private String previousHash;
private String data;
private long timeStamp;
private int nonce;
public Block(String data, String previousHash, long timeStamp) {
this.data = data;
this.previousHash = previousHash;
this.timeStamp = timeStamp;
this.hash = calculateHash();
}
public String calculateHash() {
String dataToHash = previousHash
+ Long.toString(timeStamp)
+ Integer.toString(nonce)
+ data;
MessageDigest digest = null;
byte[] bytes = null;
try {
digest = MessageDigest.getInstance("SHA-256");
bytes = digest.digest(dataToHash.getBytes(UTF_8));
} catch (NoSuchAlgorithmException ex) {
logger.info(ex.getMessage(), Level.SEVERE);
}
StringBuffer buffer = new StringBuffer();
for (byte b : bytes) {
buffer.append(String.format("%02x", b));
}
return buffer.toString();
}
public String mineBlock(int prefix) {
String prefixString = new String(new char[prefix]).replace('\0', '0');
while (!hash.substring(0, prefix).equals(prefixString)) {
nonce++;
hash = calculateHash();
}
return hash;
}
}
Block
As we said every Block has a hash. A value has unique hash and hashing is irreversible operation in cryptography, meaning it is impossible to get the original value for a given hash.
A Block has previous transaction hash value, therefore forming a chain. A data consisting of a value of operation (ex. contract). T timestamp and an arbitrary value used in cryptography(nonce).
Hash
we concatenate different parts of the block to generate a hash from, then we get an instance of the SHA-256 hash function from MessageDigest. Next, we generate the hash value of our input data, which is a byte array and we transform it to a 32-digit hex number.
Mining
is a CPU intensive task of generating a hash with a specified prefix. It is done by brute force operation.
For testing our implementation we create a simple blockchain, generate hashes, mine and validate it.
We store the blockchain in an ArrayList, we have a condition that hash needs to start with ‘aa’ string.
import static org.junit.jupiter.api.Assertions.assertTrue;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import org.example.Block;
import org.junit.jupiter.api.AfterAll;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.Test;
public class BlockchainUnitTest {
public static List<Block> blockchain = new ArrayList<Block>();
public static int prefix = 2;
public static String prefixHash = new String(new char[prefix]).replace('\0', 'a');
@BeforeAll
public static void setUp() {
Block genesisBlock = new Block("Genesis Block.", "0", new Date().getTime());
genesisBlock.mineBlock(prefix);
blockchain.add(genesisBlock);
Block firstBlock = new Block("First Block.", genesisBlock.getHash(), new Date().getTime());
firstBlock.mineBlock(prefix);
blockchain.add(firstBlock);
}
@Test
public void addNewBlockTest() {
Block newBlock = new Block("New Block.", blockchain.get(blockchain.size() - 1)
.getHash(), new Date().getTime());
newBlock.mineBlock(prefix);
assertTrue(newBlock.getHash()
.substring(0, prefix)
.equals(prefixHash));
blockchain.add(newBlock);
}
@Test
public void validateBlockchain() {
boolean condition = true;
for (int i = 0; i < blockchain.size(); i++) {
String previousHash = i == 0 ? "0"
: blockchain.get(i - 1)
.getHash();
condition = blockchain.get(i)
.getHash()
.equals(blockchain.get(i)
.calculateHash())
&& previousHash.equals(blockchain.get(i)
.getPreviousHash())
&& blockchain.get(i)
.getHash()
.substring(0, prefix)
.equals(prefixHash);
if (!condition)
break;
}
assertTrue(condition);
}
@AfterAll
public static void clear() {
blockchain.clear();
}
}
In the setup methods we create first block of the blockchain (First block of a blockchain is called genesis block). Then we add the first block.
In the new block testing method we create and mine a new block and test if calculated hash has predefined prefix of ‘aa’.
In the blockchain validation test method, we iterate through it and calculate hash for each block and check if fulfils the conditions for previous hash and predefined prefix.
Conclusion
In this blog post we explored basics of blockchain technology, went over basic tools used fr it, reviewed some Java libraries and created a sample blockchain in Java language.